Loading [MathJax]/extensions/ams.js
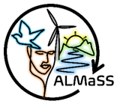 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
28 #ifndef PesticideToxicityH
29 #define PesticideToxicityH
48 virtual void reset(
void);
58 virtual void tick(
int a_step_counter_in_a_day);
87 void doToxicity(
double a_mass,
double a_para_index);
97 virtual void tick(
int a_step_counter_in_a_day,
double a_mass=1,
double a_para_index=0);
108 virtual void reset(
void);
115 #endif //end PesticideToxicityH
void doToxicity(double a_mass, double a_para_index)
The default function to calculate the toxicity effect.
Definition: PesticideToxicity.cpp:122
int Supply_m_Location_x() const
Returns the ALMaSS x-coordinate.
Definition: PopulationManager.h:239
PlantProtectionProducts
A list ofPPP names for tracking by the Pesticide class.
Definition: LandscapeFarmingEnums.h:1077
double g_rand_uni_fnc()
Definition: ALMaSS_Random.cpp:56
std::vector< double > supplyPesticideVector(void)
The function to return the pesticide amount array.
Definition: PesticideToxicity.h:99
TAnimal * m_my_animal_host
The pointer to the host animal.
Definition: PesticideToxicity.h:68
double supplyTotalPesticide(void)
The function to return the total pesticide amount.
Definition: PesticideToxicity.h:54
void doOverspray(void)
The function for the animal to be oversprayed when the pesticide application is happening where the a...
Definition: PesticideToxicity.cpp:165
Definition: PesticideToxicity.h:63
static void setLandscapePointer(Landscape *a_landscape)
The function to set the static landscape pointer.
Definition: PesticideToxicity.h:60
void addPesticide(double eating_amount, double amount_per_square, double(Landscape::*a_supply_pest_func_pointer)(int, int, PlantProtectionProducts), int loc_x, int loc_y)
The function to add pesticide based on the eating amount and the amount of food per square meter.
Definition: PesticideToxicity.cpp:79
double SupplySprayPesticideRate(int a_x, int a_y)
Gets the sprayed pesticide rate in the given location.
Definition: Landscape.cpp:1512
CfgBool l_pest_enable_pesticide_engine
Used to turn on or off the PPP functionality of ALMaSS.
void addPesticide(double a_mount, PlantProtectionProducts a_type)
The function to add pesticide based on the given amount and the given type.
Definition: PesticideToxicity.h:46
void(PesticideToxicity::* m_decay_func_pointer)(void)
The function pointer to point the function for decay calculation.
Definition: PesticideToxicity.h:76
double m_total_pest_sum
The summation of the total pesticide body burden.
Definition: PesticideToxicity.h:70
double value() const
Definition: Configurator.h:142
virtual void reset(void)
The function to reset the pesticide to zero.
Definition: PesticideToxicity.cpp:85
int m_location_previous_x
The x-coordinate is used to remember the location from the previous time-step.
Definition: PesticideToxicity.h:80
PlantProtectionProducts SupplySprayPesticideType(int a_x, int a_y)
Gets the sprayed pesticide type in the given location.
Definition: Landscape.cpp:1504
PesticideStore()
Definition: PesticideToxicity.cpp:71
void doDecay(void)
The default fuction to decay the pesticide body burden.
Definition: PesticideToxicity.cpp:91
bool value() const
Definition: Configurator.h:164
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
double SupplyPesticidePlantSurface(int a_x, int a_y, PlantProtectionProducts a_ppp)
Gets plant surface pesticide for a location.
Definition: Landscape.cpp:1444
static CfgFloat pest_animal_overspray_chance("PEST_ANIMAL_OVERSPRAY_CHANCE", CFG_CUSTOM, 0.1)
The chance being oversprayed for the host animal.
static CfgBool cfg_pest_animal_contact_on("PEST_ANIMAL_CONTACT_ON", CFG_CUSTOM, true)
The flag to turn on contact exposure path.
Bool configurator entry class.
Definition: Configurator.h:155
double supplyPestMortality(void)
The function to supply the host's mortality rate caused by pest.
Definition: PesticideToxicity.h:104
int Supply_m_Location_y() const
Returns the ALMaSS y-coordinate.
Definition: PopulationManager.h:243
The base class for all ALMaSS animal classes. Includes all the functionality required to be handled b...
Definition: PopulationManager.h:200
static CfgArray_Double pest_animal_effect_amount("PEST_ANIMAL_EFFECT_AMOUNT", CFG_CUSTOM, 1, vector< double >{2})
The pesticide burdon (mg/mg) threshold value starts to have effect on the animal.
void setHostAnimalPointer(TAnimal *animal)
The function to set the host animal pointer.
Definition: PesticideToxicity.h:106
std::vector< double > m_pest_amount_vec
The vector to store the pesticide amount for each pesticide.
Definition: PesticideToxicity.h:37
double m_pest_hazard
The hazard of the pesticide.
Definition: PesticideToxicity.h:72
virtual void tick(int a_step_counter_in_a_day)
The function to update pesticide contamination every time step.
Definition: PesticideToxicity.cpp:105
Definition: Configurator.h:208
int m_location_previous_y
The y-coordinate is used to remember the location from the previous time-step.
Definition: PesticideToxicity.h:82
CfgInt l_pest_NoPPPs
The number of active Plant Protection Products to be tracked - a performance penalty if enabled with ...
PesticideToxicity()
Definition: PesticideToxicity.cpp:113
double supplyPesticide(PlantProtectionProducts a_type)
The function to return the pesticide amount.
Definition: PesticideToxicity.h:52
int value() const
Definition: Configurator.h:116
std::vector< double > value() const
Definition: Configurator.h:219
void(PesticideToxicity::* m_toxicity_func_pointer)(double, double)
The function pointer to point the function for toxicity effect calculation.
Definition: PesticideToxicity.h:74
void SetPesticideVector(std::vector< double > a_pest_amount_vec)
Definition: PesticideToxicity.h:100
Definition: PesticideToxicity.h:33
std::vector< double > * supplyPesticide(void)
The function to return the pesticide amount array.
Definition: PesticideToxicity.h:50
void setToxicityFuncPointer(void(PesticideToxicity::*a_toxicity_func)(double, double))
The function to set the toxicity function pointer.
Definition: PesticideToxicity.h:89
void setDecayFuncPointer(void(PesticideToxicity::*a_decay_func)(void))
The function to set the decay function pointer.
Definition: PesticideToxicity.h:91
static Landscape * m_ALandscape
The static landscape pointer.
Definition: PesticideToxicity.h:39
virtual void reset(void)
Reset PesticideToxicity class, this should be called when the host animal is dead.
Definition: PesticideToxicity.cpp:195
Integer configurator entry class.
Definition: Configurator.h:102
Double configurator entry class.
Definition: Configurator.h:126
double m_my_animal_mortality
The mortality rate of the host animal caused by pesticide body burden.
Definition: PesticideToxicity.h:78
CfgFloat l_pest_zero_threshold
The number of pesticide types used, the maximum value is 10.
Definition: Configurator.h:70
double supplyPesticide(PlantProtectionProducts a_type=ppp_1)
The function to supply the pesticide residue for the given pesticide type, the default type is 0,...
Definition: PesticideToxicity.h:102
void doContact(void)
The function to calculate the pesticide contamination through contact.
Definition: PesticideToxicity.cpp:140
static CfgArray_Double pest_decay_rate_animal("PEST_DECAY_RATE_ANIMAL", CFG_CUSTOM, 10, vector< double >{0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9})
The decay rate of the pesticide body burden. The length of this vector maximum number of supported pe...
virtual void tick(int a_step_counter_in_a_day, double a_mass=1, double a_para_index=0)
The function to update pesticide contamination every time step.
Definition: PesticideToxicity.cpp:179
static CfgArray_Double pest_animal_killing_rate("PEST_ANIMAL_KILLING_RATE", CFG_CUSTOM, 1, vector< double >{0.01})
The pesticide killing rate constant for GUTS-SD.
static CfgFloat pest_overspray_animal_rate("PEST_OVERSPRAY_ANIMAL_RATE", CFG_CUSTOM, 0.01)
The absorption rate of the animal for overspray.
Definition: LandscapeFarmingEnums.h:1079
static CfgFloat pest_contact_animal_ratio("PEST_CONTACT_ANIMAL_RATIO", CFG_CUSTOM, 0.1)
The ratio to control how much the animal will be contaminated through contact.
double GetTotalPest(void)
Return the total pesticide.
Definition: PesticideToxicity.h:112
static CfgFloat pest_overspray_animal_surface("PEST_OVERSPRAY_ANIMAL_SURFACE", CFG_CUSTOM, 0.01)
The surface of the animal in square meters fo the calculation of overspray.
void eatingFromStorePest(PesticideStore *a_store, double a_eating_amount, double a_total_amount)
The function for eating from a store to add pesticide.
Definition: PesticideToxicity.cpp:206